Setting Default Values in GitHub Actions Workflows
Published October 20, 2023
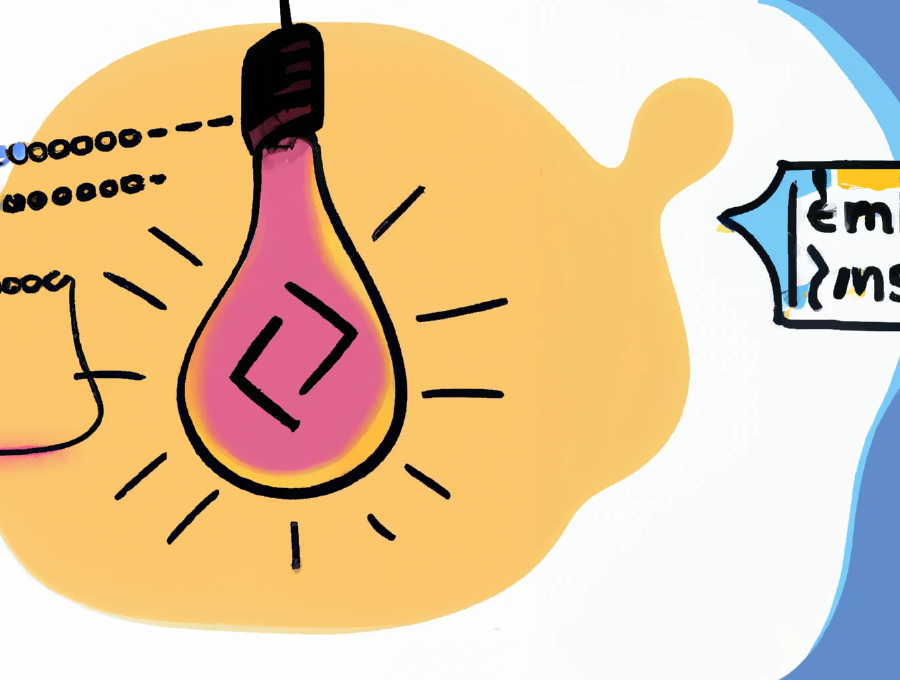
GitHub Actions is a powerful platform for automating workflows and tasks in your software development process. When creating workflows, you often need to set default values for variables or parameters, especially when certain values are not always going to be provided. In this blog post, let’s explore two techniques to set default values in GitHub Actions workflows, including a handy ! contains()
approach and the double pipe ||
hack. We’ll also discuss the potential limitations of the double pipe hack.
Setting the Stage
Imagine you have a GitHub Actions workflow that relies on input data from an external source, such as a webhook payload. You want to ensure that specific variables have default values when the payload doesn’t provide them or provides null values.
GitHub Actions supports conditional expressions that allow you to set default values based on conditions, but it’s not obvious how.
I know now of two ways of doing this, one common way to do this is by using a conditional expression (the double pipe ||
hack), however this doesn’t work with Boolean values as expected.
Method 1: Using Conditional Expressions
The double pipe ||
hack let’s you set a default value when the left side of the double pipes are empty or otherwise of a falsy value.
Here is an example workflow that takes a variable named HUE which gets a default value of “light blue” when the payload value does not provide it:
name: Using Conditional Expressions
on:
repository_dispatch:
types:
- name-of-a-webhook
jobs:
your_job_name:
runs-on: ubuntu-latest
steps:
- name: Example of using a conditional expressions to set a default
run: echo "The Hue is ${{ env.HUE }}"
env:
HUE: ${{ github.event.client_payload.data.hue || "light blue" }}
Limitations of the Double Pipe ||
Hack
While the double pipe ||
hack is a convenient way to set default values, it has its limitations:
The double pipe hack treats falsy values (i.e. undefined
, null
, false
, or an empty string) all as false
. This can lead to unexpected behaviour. For example when you provide a value of false
, and the default (intended for when the value is not provided) the outcome becomes unintentionally true
. This is because false || true
is true
.
Thus handling Boolean values is tricky, and the double pipe hack may not work as expected if you need to distinguish between false and other falsy values. In such cases, consider using the alternative method of ! contains()
.
Method 2: The ! contains()
Approach
For situations where Boolean values need to be handled differently to falsy values, you can use the ! contains()
approach:
name: The ! contains() Approach
on:
repository_dispatch:
types:
- name-of-a-webhook
jobs:
your_job_name:
runs-on: ubuntu-latest
steps:
- name: Set Default Value for ENABLED
run: echo "The value of ENABLED is ${{ env.ENABLED }}"
env:
ENABLED: ${{ !contains(github.event.client_payload.data.enabled, false) }}
In this ! contains()
approach if a value is provided and that value is false
contains method evaluates to true
and then the !
flips that back to false
so that the intended false
value is received. But if any other value or no value is provided the default of true
is correctly recived.